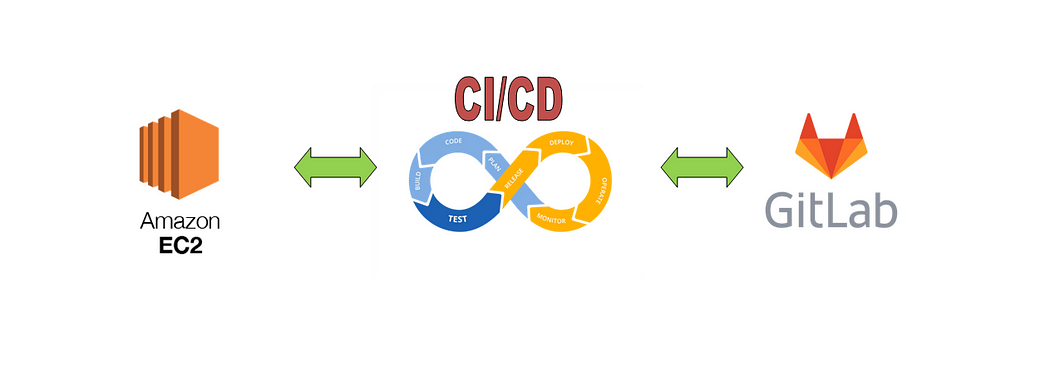
In the ever-evolving world of Information and Technology, websites remain the backbone, serving a vast audience on the internet. Many of us have created simple basic websites using HTML or utilized JavaScript utilities like React, Angular, etc. While developing such websites may seem straightforward, the challenge escalates when we aim to make our applications go live and run on the internet. There are plenty of services available on the internet that we can utilise to host our application like AWS, Netlify, Vercel, Heroku etc.
In this guide, we will be deploying our react application on AWS EC2 service. We will not only learn to deploy our application but we will also look at automating our deployment process using GItlab CI/CD. So, without any further talks
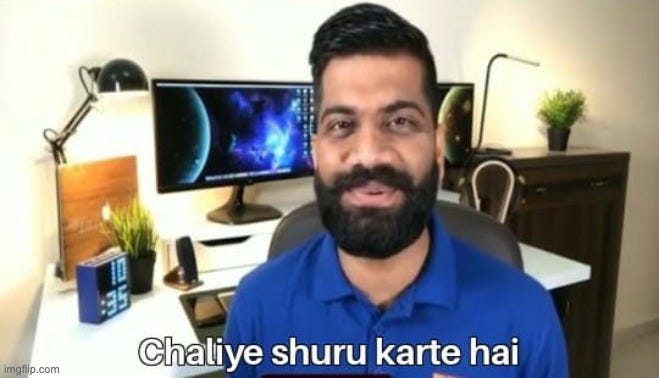
Prerequisites
1. An AWS account with appropriate permissions to create resources.
2. Basic knowledge of GitLab and CI/CD concepts.
3. Familiarity with the AWS Management Console and command-line interface (CLI).
4. Frontend react application on gitlab or you can clone this one which I will be using in this tutorial.
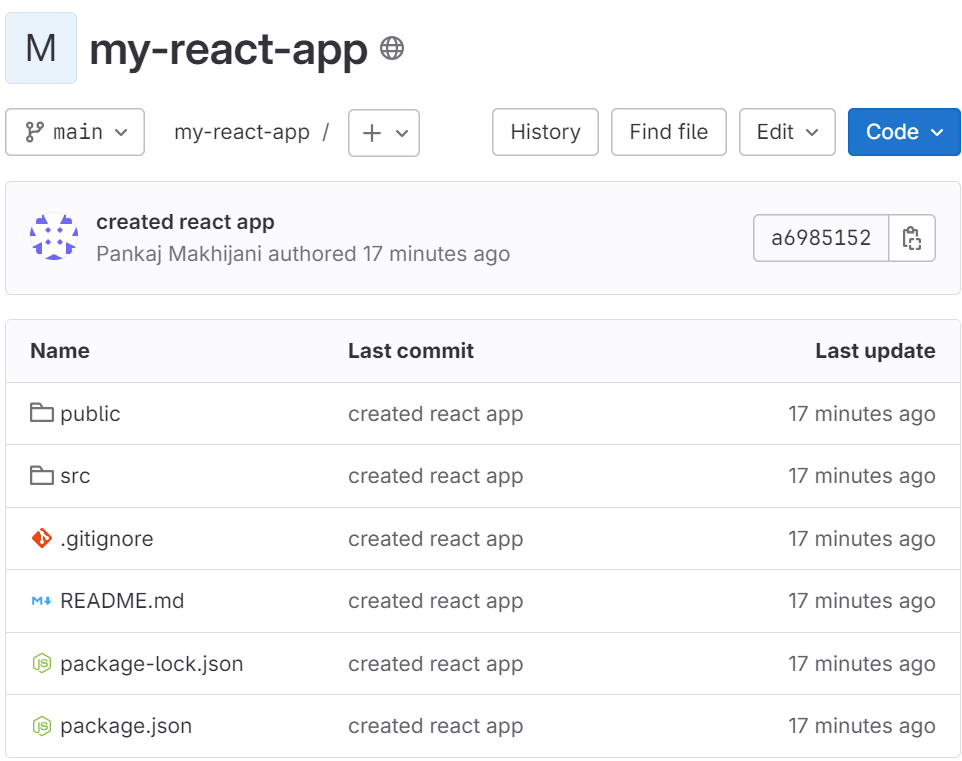
5. Gitlab runner configured with your code repository. Check my blog here for more info.
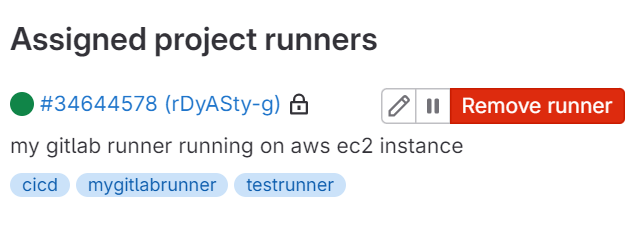
Section 1: Deploying React Application on EC2
Step 1: Setting up an EC2 instance
Here I am spinning up a new EC2 instance but you can use the same gitlab runner EC2 instance to host your react application.
- Log in to your AWS Management Console & navigate to the EC2 dashboard.
- Launch an EC2 instance, choosing an appropriate instance type and configuration.
- Ensure that the security group associated with your instance allows inbound traffic on port 80 (HTTP).

Step 2: Configure the EC2 instance
- SSH into your EC2 instance using the provided key pair.
- Update the instance by running:
sudo yum update && sudo yum upgrade -y
3. Create the required directory
sudo mkdir /var/www && sudo mkdir /var/www/html
sudo mkdir /var/www/html/reactapp
4. Install Nginx to serve react app
sudo yum install nginx
5. Configure Nginx to serve your React app:
sudo vi /etc/nginx/sites-available/reactapp
Add the following code
server {
listen 80;
server_name _;
location / {
root /var/www/html/reactapp; # Serve files from /var/www/html
index index.html;
try_files $uri $uri/ /index.html;
}
}
6. Start the nginx service
sudo systemctl start nginx
sudo systemctl enable nginx
Step 3: Upload Your Application Code
1. Ensure proper directory permissions:
sudo chown ec2-user /var/www/html && sudo chmod 755 /var/www/html
sudo chown ec2-user /var/www/html/reactapp && sudo chmod 755 /var/www/html/reactapp
2. Create a production build of your React application:
npm run build
3. Zip the build directory:
zip -r build.zip build/
4. Upload the zip file to your EC2 instance:
scp -i <YOUR_EC2_KEY_PEMFILE> build.zip ec2-user@<YOUR_EC2SERVER_IP>:/var/www/html/reactapp
5. SSH into your EC2 instance and unzip the build:
ssh -i <YOUR_EC2_KEY_PEMFILE> ec2-user@<YOUR_EC2SERVER_IP>
cd /var/www/html/reactapp
unzip build.zip
Step 4: Access Your Application
Your React app should now be accessible via your EC2 instance’s public IP address.
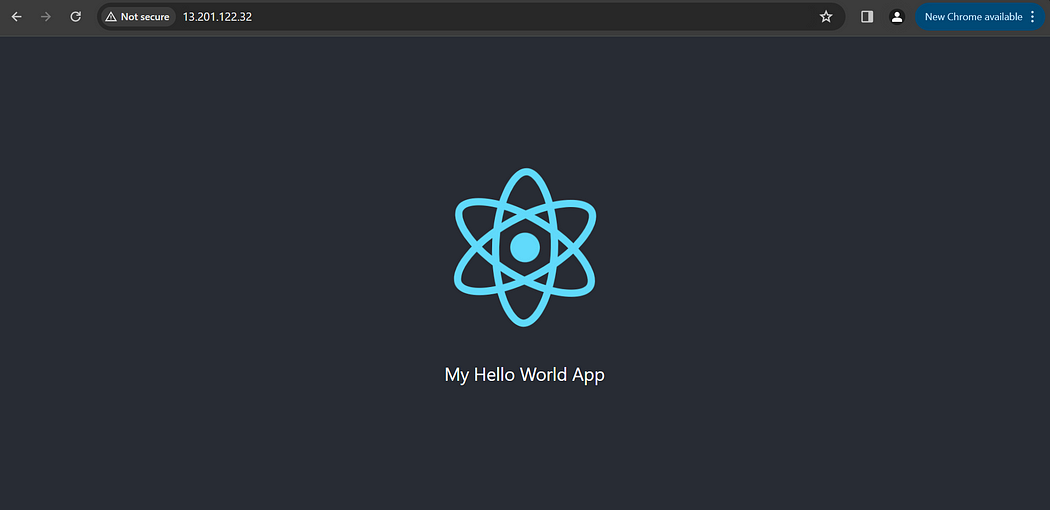
Section 2: Configuring CI/CD Pipeline
Now, let’s set up the CI/CD pipeline:
Step 3: Configuring CI/CD pipeline
Before proceeding with CICD let’s first understand the CICD workflow
- GitLab Runner triggers the pipeline upon new code commits.
- The pipeline performs the following operations:
- Installs npm dependencies.
- Builds the application.
- Zips the build directory.
- Transfers the zip file to the server.
- Removes the existing build, unzips the new build, and moves it to the appropriate directory.
The above mentioned flow seems to be quite simple and easy but while working on CICD implementation we also need to take care of our server security. Security is the major concern while working on the Production servers.
So, what we gonna do? Looking at the security side we will be creating a different SSh key for our pipeline to push code onto our server and also we will be taking advantage of gitlab CICD variables to secure our server credentials.
This might sound like a complex stuff but it’s really simple. Let’s begin
Run the below command on your app server to create a new keypair as we are going to use private key with gitlab runner
ssh-keygen -f id_rsa.gitlabrunner
Make sure you leave all passphrases empty. Just hit return when asked to enter a phrase.
This will create a id_rsa.gitlabrunner
and id_rsa.gitlabrunner.pub
. Now add the public key id_rsa.gitlabrunner.pub
to you server’s authorized keys file:
cat id_rsa.gitlabrunner.pub >> ~/.ssh/authorized_keys
Now, Inspect the new private key via cat id_rsa.gitlabrunner
-----BEGIN OPENSSH PRIVATE KEY-----
b3BlbnNzaC1rZXktdjEAAAAABG5vbmUAAAAEbm9uZQAAAAAAAAABAAABlwAAAAdzc2gtcn
NhAAAAAwEAAQAAAYEAwPtyW6gzmBfEOBRA5JYZYcPMhgbp46GyRyXkBLxEypXjBozW8dZt
ZhUrmOyXra+VOYeSz/Iewn/1KmfT7+v/DrzhmnJjKcslcSlusQ7hm4+MBwCJTOrxs2shaw
30WNtioGUZFNHOuTYrTBu+N8xTn5CHobICJNZYi1xR8EgrYw7upnTcLl+D3zmObf/eQEg7
Z/nGNnCA+uLnHApLAops09GJdEVmdGb4y8KD0DnNDMb0Hs1GYi5VQotSB5rABOK5rWPebR
URDFe7MB8ZXzvmZr95mUEWhDJDGkEStQHZXxsstr6PZSIhpkr2gyiTjcAncacFYM07+fRm
tTFfdohj+KjWkAAAAxZWMyLXVzZXJAaXAtMTAtMC0wLTkzLmFwLXNvdXRoLTEuY29tcHV0
ZS5pbnRlcm5hbAEC
-----END OPENSSH PRIVATE KEY-----
and paste the content into your gitlab CICD variables. You can find the gitlab variables under settings > CICD > variables.
- type: variable, key: EC2_HOST, value: <YOUR_APP_SERVER_IP>
- type: variable, key: EC2_USERNAME, value: ec2-user
- type: file, key: EC2_KEY, value: Your
id_rsa.gitlabrunner
file content
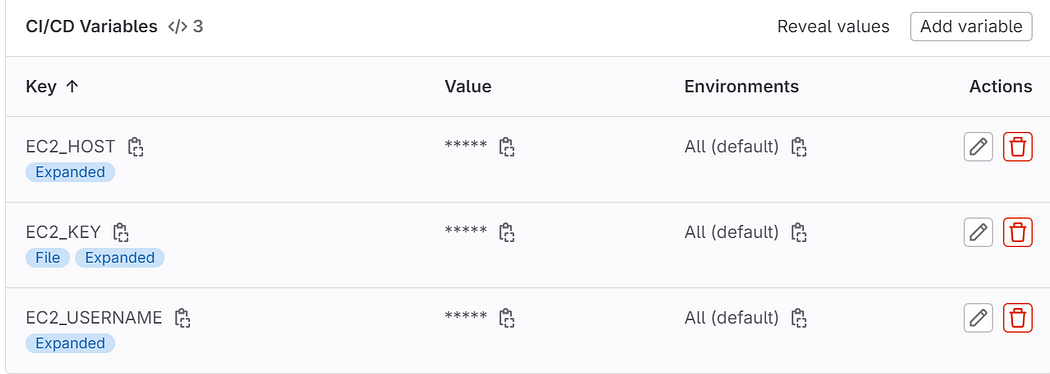
Now we are all done and now ready to implement the pipeline.
In order to integrate CICD pipeline into our project, we will create .gitlab-ci.yml file at the root of our project.
# CI/CD configuration file
# Define stages for the pipeline
stages:
- build
- deploy
# Cache configuration to speed up the build process
cache:
key: "$CI_COMMIT_REF_SLUG"
paths:
- build/ # Cache the 'build' directory
# Build stage
app_build:
stage: build
script:
- npm install # Install project dependencies
- npm run build # Build the project
- echo "Build Completed!" # Print a completion message
tags: # Tags specify the GitLab runner where this pipeline will be executed
- mygitlabrunner
only: # Define which branches trigger this pipeline stage
- main
# Deploy stage
app_deploy:
stage: deploy
script:
- |
# Change the Key Permissions
chmod 600 $EC2_KEY
# Zip the build directory
zip -r build.zip build/
# Transfer the zip file via SCP
scp -v -o StrictHostKeyChecking=no -i $EC2_KEY build.zip $EC2_USERNAME@EC2_HOST:/var/www/html
# SSH into the remote server and unzip the file
ssh -o StrictHostKeyChecking=no -i $EC2_KEY $EC2_USERNAME@$EC2_HOST "rm -vrf /var/www/html/reactapp/* && cd /var/www/html/reactqpp && mv /var/www/html/build.zip . && unzip -o build.zip && mv build/* ."
# Remove the zip file after transfer
rm build.zip
echo "Deployment Completed!" # Print a completion message
tags: # Tags specify the GitLab runner where this pipeline will be executed
- mygitlabrunner
only: # Define which branches trigger this pipeline stage
- main
After adding the above gitlab-ci file at the root of your project. Now let’s go and push some changes into our app.js file to check our CICD implementation.
Once you push the changes, you will notice that your pipeline execution has begun.

Wait for the time till it completes the pipeline execution and you will see that your application is now deployed.
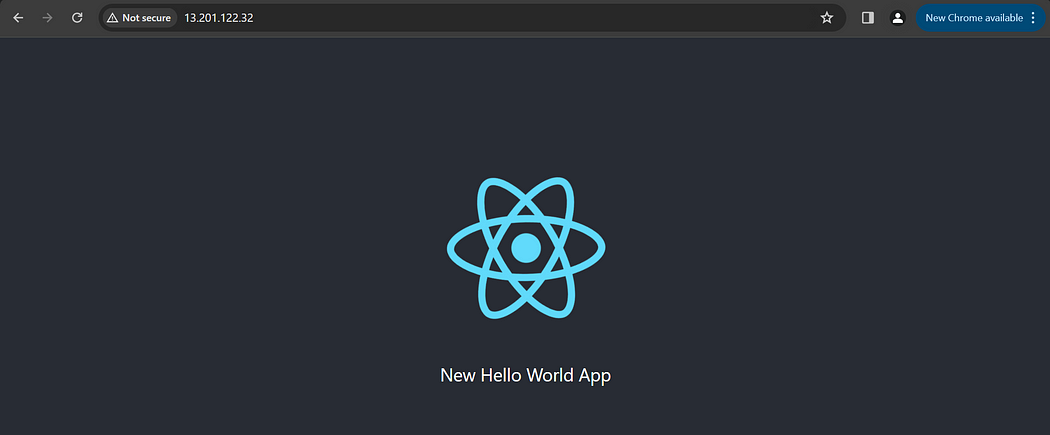
Wohooo.. your gitlab CICD pipeline is now successfully setup 🥳
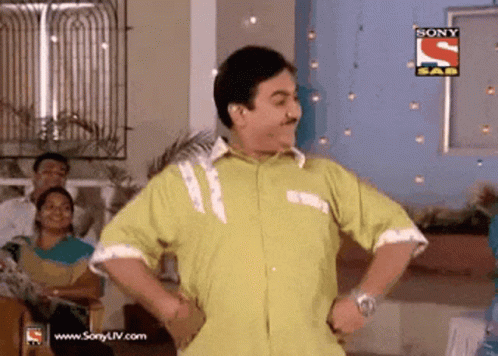
Conclusion
In this blog, we have understood and successfully set up GitLab CICD pipeline to deploy our frontend react app onto an AWS EC2 instance. This setup empowers us to streamline our development process, increase productivity, and deliver high-quality software efficiently.
I hope you found this content informative and enjoyable. For more blogs and updates, please consider following and clicking the 👏 button below to show your support. Happy coding! 🚀
Thank you for reading! 💚