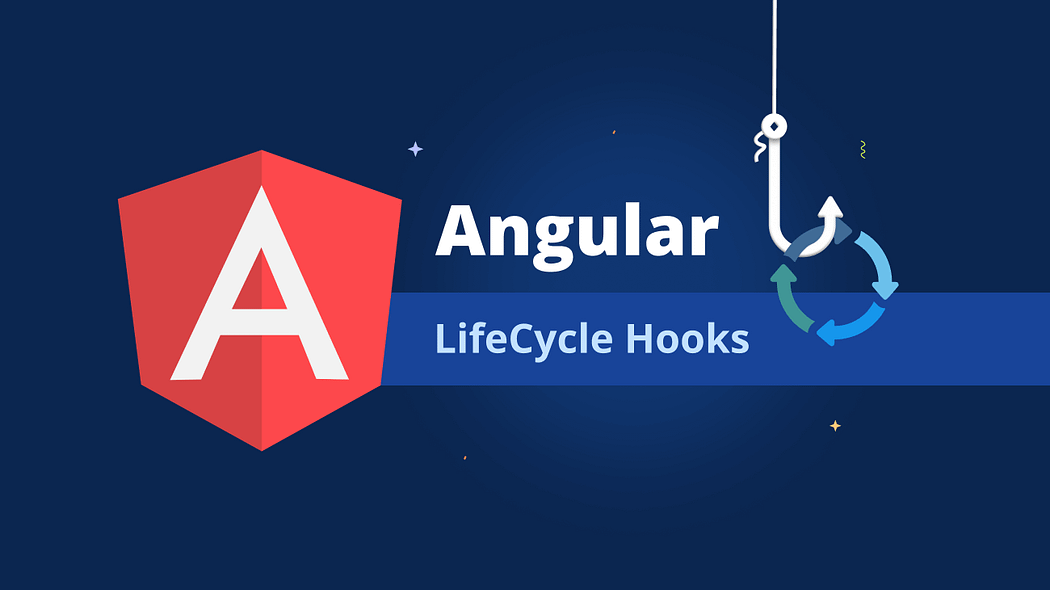
Hello all π, In this topic I have tried to cover everything related to Angular component lifecycle hook along with the Code Sample. But, before starting this make sure you are familiar with AngularJS.
Angular component lifecycle hooks
Angular is a frontend evelopment framework that is used for building single page applications. It makes of use of individual resuable components for building scalable web applications. These components follows a series of different phases from being created to being destroyed. A component lifecycle goes typically through eight different stages. We can hook into these different lifecycle phases to get more control over our application.
In order to do this, we make use of certain functions/methods which gets invoked during these lifecycle phases. These methods/functions are called hooks.
These hooks are executed in this order.
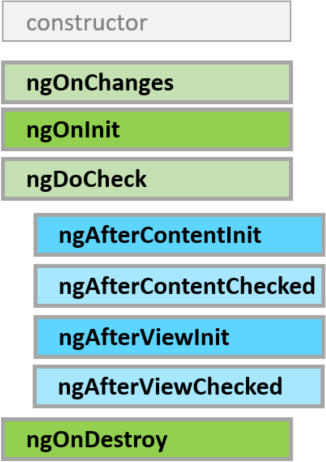
Functions in lifecycle hooks
The components in angular are typescript classes that is why every component must be considered as constructor method. The the lifecyce hook methods, constructor gets executed first and then only executiong of lifecycle methods takes place.
ngOnChanges
Purpose
β It responds when angular sets or resets data bound input properties.
β Tracks current and previous values.
β Only tracks @Input property values.
Timing
β Called before ngOnInit() and whenever the input changes.
Limitations
β Fails to detect changes user object types.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements OnChanges {
@Input() prop: number = 0;
ngOnChanges(changes: SimpleChanges) {
// changes.prop contains the old and the new value...
}
}
ngOnInit
Purpose
β It responds when angular first displays the data bound properties.
β To Perform initialization shortly after constructor.
β To setup the component after angular sets the input properties.
β One-and-Done Hook!
Timing
β Called only once after the first ngOnChanges()
Limitations
β A directiveβs input properties are not set until constructor()
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements OnInit {
ngOnInit() {
// ...
}
}
ngDoCheck
Purpose
β Detect when angular doesnβt detects on its own.
β Compares currents state against previous values
Timing
β Called during every change detection run.
β Called immediately after ngOnChanges() and ngOnInit()
Limitations
β Called with an enormous frequency.
β Dependency must be very less or the user experience suffers.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements DoCheck {
ngDoCheck() {
// ...
}
}
ngAfterContentInit
Purpose
β Respond when angular projects external content into the componentβs view.
β To perform additional initialization tasks shortly after content projection.
Timing
β Called only once after angular fully initialized all the contents.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements AfterContentInit {
ngAfterContentInit() {
// ...
}
}
ngAfterContentChecked
Purpose
β It responds when change detector completes the content checking.
β To perform tasks after every content checking.
Timing
β Called after ngAfterContentInit() and every subsequent ngDoCheck().
Limitations
β Instantiated before the changes in child component.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements AfterContentChecked {
ngAfterContentChecked() {
// ...
}
}
ngAfterViewInit
Purpose
β It responds when angular fully initialized a componentβs view.
β To perform tasks after all set in the view.
Timing
β Called once after the first ngAfterContentChecked().
Limitations
β Invoked only once after the view is initialized.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements AfterViewInit {
ngAfterViewInit() {
// ...
}
}
ngAfterViewChecked
Purpose
β It responds after Angular checks the componentβs views and child views, or the view that contains the directive..
β To perform tasks after every content checking.
Timing
β Called after the ngAfterViewInit() and every subsequent ngAfterContentChecked().
Limitations
β Called even when there is no changes so performance issues.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements AfterViewChecked {
ngAfterViewChecked() {
// ...
}
}
ngOnDestroy
Purpose
β Clean just before angular destroys the component.
β Time to notify the other components.
β Unsubscribe observables, detach DOM events, stop interval timers, free up the memory leaks and resources.
Timing
β Called just before angular destroys the component.
Limitations
β Instantiated before the changes in child component.
Code Sample
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements OnDestroy {
ngOnDestroy() {
// ...
}
}
Conclusion
I hope this tutorial was helpful for you and you have understood different functions of angular components life cycle. Thank you for reading, have a nice day π